May 1, 2024
Understanding the JavaScript Spread Operator
Justin Golden
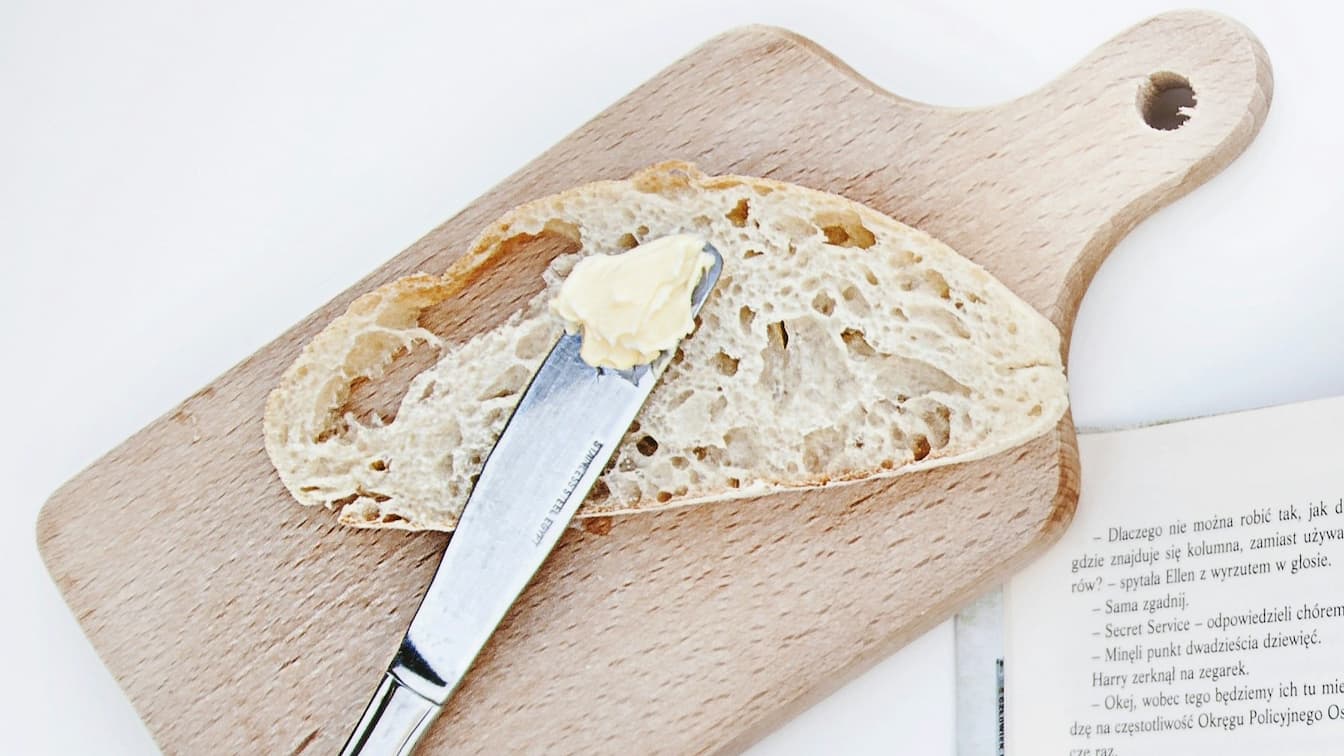
The spread operator (...
) in JavaScript is a powerful syntax for expanding iterables like arrays or objects into individual elements. It simplifies working with data structures, enabling various operations such as copying, merging, and destructuring.
Copying and Merging Arrays and Objects
Let’s explore how the spread operator works in simple use cases with arrays and objects
Examples:
- Copying Arrays:
Create a copy of an array without modifying the original
const originalArray = [1, 2, 3];
const copiedArray = [...originalArray];
Explanation: In this example, originalArray
contains [1, 2, 3]
. By using the spread operator (...
), we create a new array copiedArray
that contains the same elements as originalArray
. This creates a shallow copy, ensuring that changes to copiedArray
do not affect originalArray
.
- Merging Arrays:
Combine two arrays into one without changing the original
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const mergedArray = [...array1, ...array2];
Explanation: Here, we have two arrays, array1
and array2
. By using the spread operator, we merge them into a single array mergedArray
. This concatenates the elements of array1
followed by the elements of array2
.
- Copying Objects:
Duplicate an object’s properties into a new object
const originalObject = { name: 'John', age: 30 };
const copiedObject = { ...originalObject };
Explanation: In this example, originalObject
contains { name: 'John', age: 30 }
. By spreading originalObject
, we create a new object copiedObject
with the same key-value pairs. Similar to array copying, this creates a shallow copy of the object.
- Merging Objects:
Combine properties from multiple objects into one.
const object1 = { name: 'John' };
const object2 = { age: 30 };
const mergedObject = { ...object1, ...object2 };
Explanation: Here, we have two objects, object1
and object2
. Using the spread operator, we merge them into a single object mergedObject
. If there are duplicate keys, the values from the later objects override the values from the earlier objects.
Using the Spread Operator to Map Data
Let’s dissect three examples of .map()
methods to understand why each approach is useful.
We will define an example object, people
:
const people = [
{
id: '1',
name: 'Alice',
age: 30,
city: 'New York',
country: 'USA'
},
{
id: '2',
name: 'Bob',
age: 25,
city: 'London',
country: 'UK'
},
{
id: '3',
name: 'Charlie',
age: 35,
city: 'Sydney',
country: 'Australia'
}
];
1. Using data
Object
people.map((person) => ({
name: person.name,
type: 'person',
data: { ...person } // Create a new object with all properties of the person
}));
Explanation:
Here, the spread operator { ...person }
is used to create a new object within the data
property. This approach maintains the original structure of the person
object intact while encapsulating all its properties within the data
key. This method can be useful when you want to organize and encapsulate data for clarity or to avoid potential naming conflicts.
2. Spreading Directly
people.map((person) => ({
name: person.name,
type: 'person',
...person // Spread all properties of the person directly
}));
Explanation:
In this example, the spread operator is applied directly to the person
object without encapsulating it within another object. This approach spreads all properties of the person
object directly into the new object being created. It’s concise and efficient, making it suitable for scenarios where you want to flatten the object structure and keep the resulting objects lightweight.
3. Destructuring and Spreading
.map(person => {
const { id, name, ...rest } = person;
return {
id,
name,
type: 'person',
...rest // Spread the rest of the properties directly
};
});
Explanation:
Here, the spread operator is used in conjunction with object destructuring. The properties id
, brn
, name
, and status
are explicitly extracted from the person
object, while the remaining properties are collected into the rest
object using the rest syntax ...rest
. This method offers fine-grained control over which properties to include in the new object, making it useful when you need to selectively include or exclude certain properties.
Summary
In summary, the JavaScript spread operator (...
) simplifies working with arrays and objects by enabling copying, merging, and spreading elements into function arguments. It’s versatile for encapsulating data, flattening object structures, and selectively including properties, making it valuable in modern JavaScript development.
More Blog Articles