May 1, 2024
A Beginner's Guide to JSON
Justin Golden
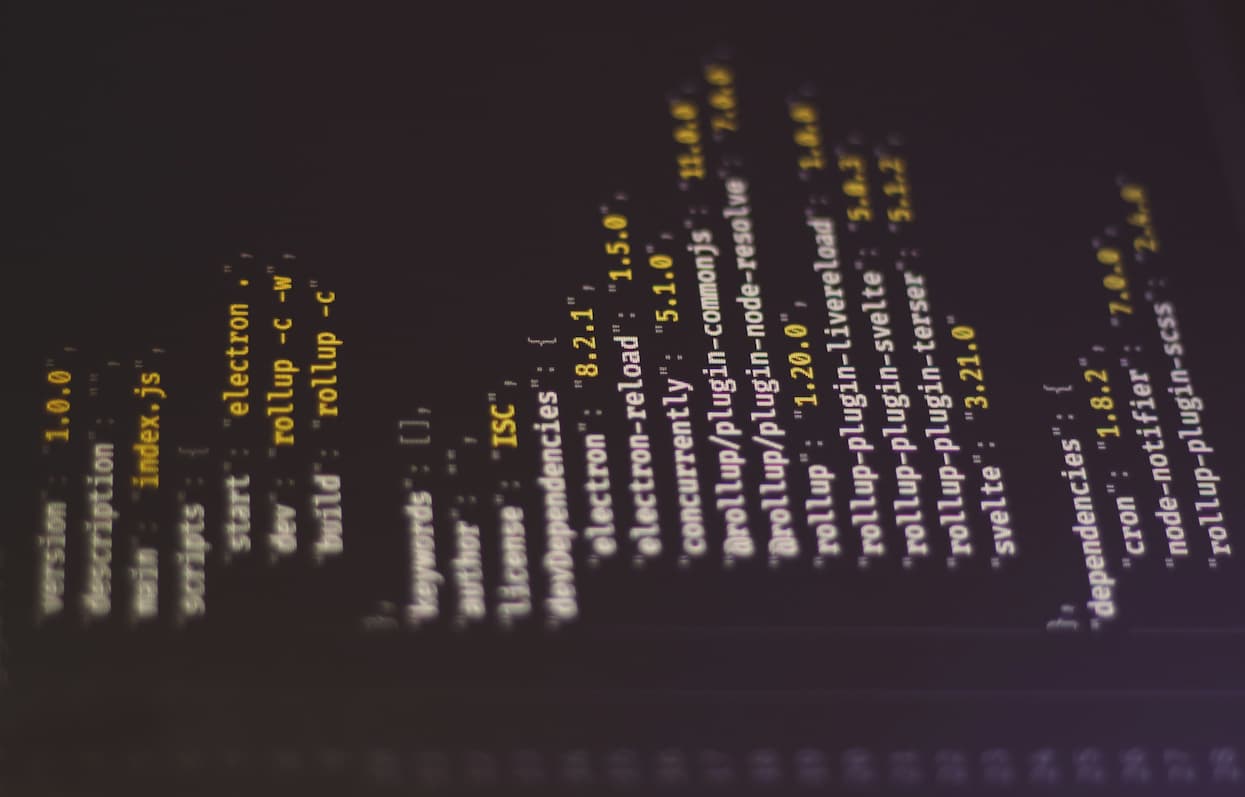
If you’re new to programming and JavaScript, you may have heard about something called JSON. But what exactly is JSON, and why is it important? Let’s break it down in simple terms.
What is JSON?
JSON stands for JavaScript Object Notation. It’s a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. In simple terms, JSON is a way to represent data.
Web APIs often return data in JSON format, making it very common on the web and a good format to use and understand. JSON is also used within JavaScript itself, which is the scripting language of the web.
How does JSON work?
JSON uses a simple syntax to represent data. It consists of key-value pairs, where the key is like a label and the value is the actual data. Values can be strings, numbers, arrays (lists of values), objects (collections of key-value pairs), booleans (true or false), or null (empty value).
For example:
{
"name": "John",
"age": 30,
"isStudent": true,
"favoriteBooks": ["Harry Potter", "The Hobbit"],
"address": {
"city": "New York",
"country": "USA"
}
}
In this JSON:
"name"
is a string key with the value"John"
."age"
is a number key with the value30
."isStudent"
is a boolean key with the valuetrue
."favoriteBooks"
is an array key with an array value containing strings."address"
is an object key with an object value containing more key-value pairs.
Commas are used to separate key-value pairs, and curly braces {}
are used to denote objects. Square brackets []
are used to denote arrays.
Why is JSON important in JavaScript?
In JavaScript, objects are represented in a similar way to JSON. JSON is commonly used as a data format in web development because it’s easy to understand and work with. When working with web servers, data is often exchanged in JSON format. JSON files typically have a .json
extension and are used for storing and transmitting data between systems.
How to use JSON in JavaScript
In JavaScript, you can work with JSON using built-in functions like JSON.parse()
and JSON.stringify()
.
JSON.parse()
is used to convert a JSON string into a JavaScript object.JSON.stringify()
is used to convert a JavaScript object into a JSON string.
For example:
const jsonString = '{"name": "John", "age": 30}';
const person = JSON.parse(jsonString);
console.log(person.name); // Output: John
const personObject = { name: 'Alice', age: 25 };
const jsonObject = JSON.stringify(personObject);
console.log(jsonObject); // Output: {"name":"Alice","age":25}
With these functions, you can easily work with JSON data in your JavaScript code.
Commas and Quotes in JSON
In JSON, commas and quotes are essential for organizing data and specifying string values.
1. Commas:
- Commas separate elements within arrays and key-value pairs within objects. They ensure clear separation between different parts of the data.
- For example,
[1, 2, 3]
uses commas to separate each number, and{"name": "John", "age": 30}
uses commas between each key-value pair.
2. Quotes:
- Quotes (specifically double quotes) enclose string values in JSON. They indicate that the content is a string and help distinguish it from other data types.
- Strings in JSON must always be enclosed in double quotes to be valid. Using single quotes or backticks is not allowed.
- For instance,
"name": "John"
shows a string value"John"
for the key"name"
.
JSON Specifics:
- JSON does not allow trailing commas, meaning you can’t have a comma after the last element in an array or object.
- Valid JSON strictly adheres to the syntax rules, ensuring compatibility across different systems.
JavaScript Extras:
- In JavaScript, you can use different quotes (single, double, or backticks) for strings and you can use trailing commas in arrays and objects
- JavaScript allows comments, which are not part of the JSON syntax
- JavaScript allows you to not use quotes for the key names if possible (i.e., contains no spaces or dashes, etc.)
Understanding commas and quotes in JSON is crucial for creating valid JSON data that can be accurately parsed by systems. While JSON has strict syntax rules, JavaScript offers more flexibility, allowing for additional features like different quotes, trailing commas, and comments in code.
JSON Best Practices
Valid Syntax:
- Ensure your JSON data follows the correct syntax, including using double quotes for strings and avoiding trailing commas.
Data Validation:
- Validate JSON data to ensure it meets expected format and structure, preventing errors in your application.
Consistent Formatting:
- Maintain consistent formatting for improved readability and easier debugging.
Error Handling:
- Implement robust error handling to manage parsing errors and provide clear error messages.
Limitations of JSON
No Comments:
- JSON does not support comments within the data.
Limited Data Types:
- JSON supports a limited set of data types and does not handle more complex types like functions or dates.
No Circular References:
- JSON cannot handle circular references, which can cause parsing issues.
Cross-Domain Requests:
- JSON data retrieval from different domains requires server support for Cross-Origin Resource Sharing (CORS) or JSONP.
Alternatives to JSON
- YAML (YAML Ain’t Markup Language):
- Known for its human-readable format and support for complex data structures, often used in configuration files and data serialization tasks.
- CSV (Comma-Separated Values):
- CSV is a plain text format used for storing tabular data, commonly used in spreadsheets, databases, and data interchange between different software applications. Each line represents a record, and fields within each record are separated by commas or other delimiters.
- XML (eXtensible Markup Language):
- Used in scenarios requiring hierarchical data representation, such as web services and configuration files.
- Protocol Buffers:
- Developed by Google, are a compact and efficient mechanism for serializing structured data. They are widely used in distributed systems and APIs for efficient serialization and deserialization.
- MessagePack:
- Offers compact binary serialization, making it ideal for high-performance applications and network protocols. It efficiently packs data into a binary format, resulting in smaller message sizes compared to JSON or XML.
Exploring these alternatives can provide tailored solutions for specific requirements beyond what JSON offers, ensuring flexibility and efficiency in data management and exchange.
Conclusion
JSON is a simple and widely used format for representing and exchanging data. Understanding JSON is essential for anyone working with web development, as it’s commonly used in building web applications. With its simplicity and versatility, JSON is a valuable tool for programmers of all levels.
More Blog Articles