September 16, 2022
A Simple Guide to Redirects in Svelte Kit
Justin Golden
The Problem
You want to handle redirects without creating a new page or a lot of code for each new redirect. You want something that works and that scales.
The Solution
Create a hooks.server.js
file in your src
directory:
import { redirect, type Handle } from '@sveltejs/kit';
const redirects = {
'/test1': '/test2'
};
export const handle: Handle = async ({ event, resolve }) => {
if (redirects[event.url.pathname]) throw redirect(301, redirects[event.url.pathname]);
return await resolve(event);
};
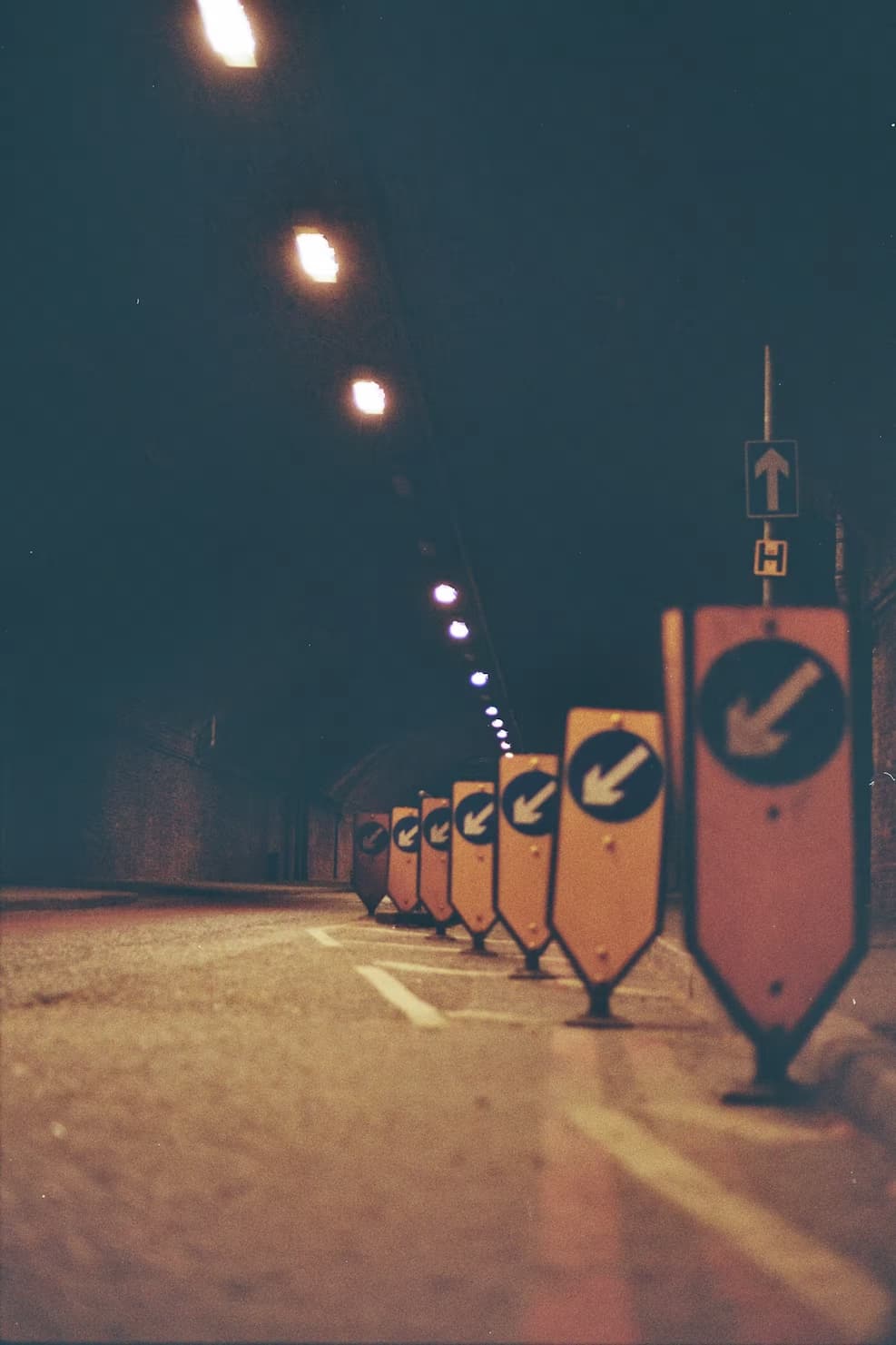
The Details
We first define a mapping of
redirects
which will direct from the key (eg./test1
) to the value (eg./test2
).We export a
handle
function, which takes in theevent
which represents the request and aresolve
function which generates a response (in our case, rendering a page, see the docs):
export async function handle({ event, resolve }) {
- We then check to see if
redirects
contains the current page (event.url.pathname
):
if (redirects[event.url.pathname])
- If the redirect exists, then we return a response with status 301 (permanent redirect, 302 is temporary, Status code 301) to the new location,
redirects[event.url.pathname])
throw redirect(301, redirects[event.url.pathname]);
Basically, the code would check if redirects
contains that key and if so redirect to the value, so if the value exists, redirect to it:
if ('/test2') throw redirect(301, '/test2');
- If there is no redirect, then we simply resolve the event and return that response, not changing anything from default behavior.
return await resolve(event);
Thank you KTibow for helping update this article to the latest version of SvelteKit. Updated 07/20/2023
More Blog Articles